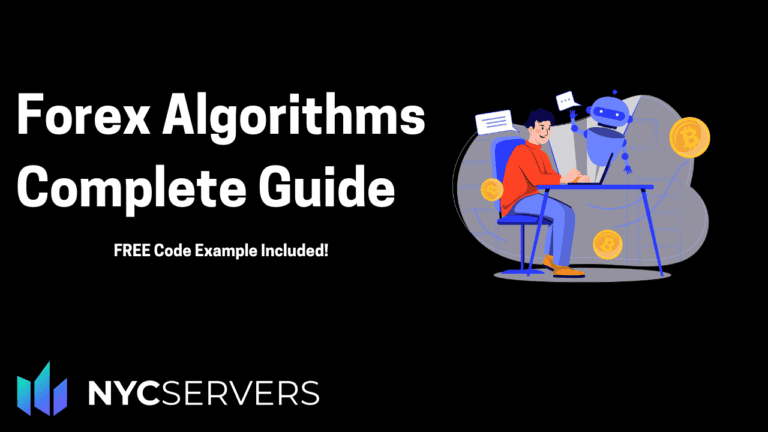
The currency markets run 24/7, processing over $6.6 trillion in daily trades. In this high-speed environment, algorithms have become essential tools for traders seeking consistent profits. Modern forex algorithms combine advanced programming with sophisticated trading strategies to automate currency trading, allowing both seasoned programmers and beginners to harness powerful tools for more efficient and profitable trading.
This comprehensive guide covers everything from basic concepts to advanced implementation, with practical examples and step-by-step instructions to help you develop, test, and deploy profitable trading algorithms in today’s dynamic currency markets.
Table of Contents
Understanding Forex Algorithms: Core Concepts
What Makes Forex Algorithms Different?
Currency markets present unique challenges and opportunities for algorithmic trading. Unlike stock markets, forex operates 24 hours a day, experiences varying levels of volatility, and offers significant leverage opportunities. These characteristics make algorithms particularly valuable for forex traders.
A forex algorithm is essentially a set of programmed rules that:
- Analyzes market conditions using predefined parameters
- Identifies trading opportunities based on your strategy
- Executes trades automatically when conditions are met
- Manages positions according to risk parameters
- Monitors and adjusts to changing market conditions
Key Components of Forex Algorithms
Modern trading algorithms consist of several crucial elements:
- Data Processing Engine
- Handles real-time market data feeds
- Processes technical indicators
- Manages historical data for analysis
- Strategy Implementation
- Converts trading rules into executable code
- Implements entry and exit conditions
- Manages position sizing and risk parameters
- Execution Module
- Connects to trading platforms
- Handles order routing
- Manages trade execution
- Risk Management System
- Monitors exposure levels
- Implements stop-loss and take-profit orders
- Ensures compliance with risk parameters
Types of Trading Algorithms: From Simple to Complex
Basic Algorithmic Strategies
- Trend Following
- Moving average crossovers
- Breakout systems
- Momentum indicators
- Mean Reversion
- Currency pair oscillation tracking
- Overbought/oversold indicators
- Statistical arbitrage
- Breakout Detection
- Volume-based breakouts
- Price pattern recognition
- Volatility breakout systems
Advanced Algorithm Categories
1. Machine Learning Based
Modern forex algorithms increasingly incorporate machine learning techniques:
- Neural networks for pattern recognition
- Support vector machines for trend prediction
- Random forests for market regime classification
2. High-Frequency Trading (HFT)
These sophisticated algorithms capitalize on minimal price movements:
- Ultra-low latency execution
- Tick data analysis
- Microstructure trading strategies
3. Hybrid Systems
Combining multiple approaches for robust performance:
- Multi-timeframe analysis
- Cross-market correlation tracking
- Adaptive parameter adjustment
Programming Your First Forex Algorithm
Basic Algorithm Example (MQL5)
Let’s build a practical forex trading algorithm that even beginners can understand and modify. This example implements a moving average crossover strategy, one of the most popular approaches in forex trading. We’ll break down each component to understand how it works.
First, let’s look at the core structure:
//+------------------------------------------------------------------+
//| MA_Crossover_EA.mq5 |
//| Copyright 2025 |
//+------------------------------------------------------------------+
#property copyright "Copyright 2025"
#property version "1.00"
#property strict
// Input Parameters
input int FastMA_Period = 10; // Fast Moving Average Period
input int SlowMA_Period = 21; // Slow Moving Average Period
input double RiskPercent = 1.0; // Risk per trade (%)
input double TakeProfit = 100; // Take Profit in points
input double StopLoss = 50; // Stop Loss in points
This initial setup defines our expert advisor (EA) properties and input parameters. Traders can adjust these parameters directly in MetaTrader 5 without modifying the code. The input
variables allow for easy strategy customization.
Next, let’s look at how we initialize our indicators:
// Global Variables
int fastMA_handle; // Fast MA indicator handle
int slowMA_handle; // Slow MA indicator handle
double fastMA[]; // Fast MA buffer array
double slowMA[]; // Slow MA buffer array
int OnInit()
{
// Initialize Moving Averages
fastMA_handle = iMA(_Symbol, PERIOD_CURRENT, FastMA_Period, 0, MODE_EMA, PRICE_CLOSE);
slowMA_handle = iMA(_Symbol, PERIOD_CURRENT, SlowMA_Period, 0, MODE_EMA, PRICE_CLOSE);
// Set arrays as series
ArraySetAsSeries(fastMA, true);
ArraySetAsSeries(slowMA, true);
return(INIT_SUCCEEDED);
}
The initialization function sets up our moving averages. The iMA()
function creates handles for our indicators, which we’ll use to access the moving average values. Setting arrays as series means the most recent data will be at index 0, making our code more intuitive.
Here’s the heart of our trading logic:
void OnTick()
{
// Check if we have enough bars to trade
if(Bars(_Symbol, PERIOD_CURRENT) < SlowMA_Period) return;
// Copy MA values
if(CopyBuffer(fastMA_handle, 0, 0, 3, fastMA) <= 0) return;
if(CopyBuffer(slowMA_handle, 0, 0, 3, slowMA) <= 0) return;
// Check for open positions
if(PositionsTotal() == 0)
{
// Calculate lot size
double lotSize = CalculateLotSize(StopLoss);
// Check for cross up (buy signal)
if(fastMA[1] <= slowMA[1] && fastMA[0] > slowMA[0])
{
OpenBuy(lotSize);
}
// Check for cross down (sell signal)
else if(fastMA[1] >= slowMA[1] && fastMA[0] < slowMA[0])
{
OpenSell(lotSize);
}
}
}
This function runs on every tick and implements our trading strategy:
- First, it verifies we have enough price data
- Then it gets the latest moving average values
- If we have no open positions, it looks for crossing signals
- When a cross occurs, it opens a trade with proper position sizing
The position sizing calculation is handled by a separate function:
double CalculateLotSize(double stopLossPoints)
{
double accountBalance = AccountInfoDouble(ACCOUNT_BALANCE);
double riskAmount = accountBalance * (RiskPercent / 100);
double tickSize = SymbolInfoDouble(_Symbol, SYMBOL_TRADE_TICK_SIZE);
double tickValue = SymbolInfoDouble(_Symbol, SYMBOL_TRADE_TICK_VALUE);
double pointValue = tickValue / tickSize;
double lotSize = NormalizeDouble(riskAmount / (stopLossPoints * pointValue), 2);
// Check minimum and maximum lot sizes
double minLot = SymbolInfoDouble(_Symbol, SYMBOL_VOLUME_MIN);
double maxLot = SymbolInfoDouble(_Symbol, SYMBOL_VOLUME_MAX);
lotSize = MathMin(MathMax(lotSize, minLot), maxLot);
return lotSize;
}
This function implements proper risk management by:
- Calculating how much money we’re willing to risk based on our account balance
- Converting that into an appropriate position size
- Ensuring the position size meets broker requirements
Advanced Features to Add
https://www.babypips.com/learn/forex/trade-management-rulesBuilding upon our basic moving average algorithm, several advanced features can significantly improve its performance and reliability. Here’s what you can incorporate into your trading system:
Risk Management Suite
A comprehensive risk management system is essential for long-term success. This includes daily loss limits that automatically halt trading when reached, preventing catastrophic losses. Implement a maximum drawdown monitor that tracks your account’s equity curve and stops trading if the drawdown exceeds predetermined levels. Position sizing should adapt dynamically to market volatility and account balance changes, ensuring consistent risk across all trades.
Market Analysis Tools
Enhance your algorithm’s market awareness by incorporating volatility filters. Use the Average True Range (ATR) indicator to measure market volatility and adjust position sizes accordingly. Implement spread monitoring to avoid trading during unfavorable market conditions when costs are too high. Add multiple timeframe analysis to confirm trends across different time horizons, providing more reliable trading signals.
Trade Management System
Develop a sophisticated trade management approach that includes trailing stops to protect profits while letting winners run. Implement a break-even feature that moves your stop loss to entry price once trades reach a certain profit level. Add partial profit taking rules that scale out of positions gradually, securing gains while maintaining exposure to further market moves.
Performance Monitoring
Create a robust system for tracking your algorithm’s performance. This includes monitoring key metrics such as:
- Win rate and profit factor
- Average win/loss ratio
- Maximum drawdown
- Trade duration statistics
- Risk-adjusted returns
Market Regime Detection
Incorporate tools to identify different market conditions (trending, ranging, or volatile) and adjust your strategy accordingly. This adaptive approach helps your algorithm perform consistently across various market environments rather than just in specific conditions.
Safety Features
Add protective measures such as:
- News event filters to avoid trading during high-impact announcements
- Slippage protection to ensure trades execute within acceptable price ranges
- Maximum spread filters to avoid trading in costly market conditions
- Connection monitoring to handle platform or internet disruptions safely
These advanced features transform a basic trading algorithm into a robust trading system capable of handling real-market challenges while protecting your capital.
What Percentage of Forex Trading is Algorithmic?
The forex market has seen a dramatic shift toward algorithmic trading in recent years. Current estimates indicate that over 90% of forex trading volume is now executed through algorithms. Institutional traders lead this trend, with major banks and hedge funds running sophisticated algorithmic trading operations. Retail traders are increasingly adopting algorithmic approaches as well, thanks to more accessible trading platforms and programming tools.
How Profitable is Algorithmic Trading?
Profitability in algorithmic forex trading varies significantly based on strategy sophistication and market conditions. While some high-frequency trading firms report annual returns exceeding 60%, retail algorithmic traders typically see more modest results. Notable examples include:
- Renaissance Technologies’ Medallion Fund, achieving a 71.8% average annual return through algorithmic trading
- Major banks reporting 20-30% of trading revenue from algorithmic operations
- Retail algorithmic traders commonly targeting 15-25% annual returns
However, it’s crucial to understand that these results require significant investment in technology, research, and risk management systems.
Popular Forex Algorithmic Trading Strategies
Several algorithmic strategies have proven successful in the forex market:
- Trend Following Systems
- Utilize moving averages and momentum indicators
- Focus on capturing major market moves
- Typically hold positions for several days or weeks
- Statistical Arbitrage
- Exploit price discrepancies between related currency pairs
- Require ultra-fast execution and precise timing
- Popular among institutional traders
- Mean Reversion
- Capitalize on price deviations from historical averages
- Work best in ranging market conditions
- Often combined with volatility indicators
- News-Based Algorithms
- React to economic announcements and news events
- Require sophisticated natural language processing
- Focus on short-term price movements
Choosing the Right Trading Platform
Platform selection is crucial for algorithmic trading success. Key factors to consider include:
- Execution Speed
- Low latency connections
- Reliable order execution
- Fast market data feeds
- Development Environment
- Built-in programming languages (MQL4/5, Python support)
- Debugging and testing tools
- Community support and resources
- Cost Structure
- Commission rates
- Spread pricing
- API access fees
MetaTrader 5 remains the most popular choice for retail traders due to its robust development environment and wide broker support. Institutional traders often opt for professional platforms like FIX API or custom solutions.
Conclusion
Algorithmic forex trading represents the future of currency markets, offering traders the ability to execute strategies with precision and consistency impossible to achieve manually. While the barrier to entry has lowered significantly, success requires a combination of programming skill, market knowledge, and robust risk management.
For traders considering algorithmic forex trading, start with simple strategies and gradually add complexity as you gain experience. Focus on risk management and system reliability before pursuing aggressive returns. Remember that successful algorithmic trading is a marathon, not a sprint, requiring continuous learning and adaptation to changing market conditions.
Whether you’re a retail trader looking to automate your strategy or an institutional investor seeking market advantages, algorithmic forex trading offers powerful tools to achieve your trading goals. The key is to approach it systematically, test thoroughly, and maintain realistic expectations about returns and risks.